If you’ve ever wondered what is unit testing in software testing, you’re not alone. Unit testing is the process of testing individual components of code, like functions or methods, to make sure they perform correctly on their own. It’s a simple but powerful way to catch bugs early, improve code quality, and make future updates safer. In the software development lifecycle, unit testing acts as the first line of defense, helping teams build reliable systems one piece at a time.
1. What is Unit Testing?
Unit testing is a type of software testing where individual components or functions of a program are tested in isolation to ensure they work as expected. It’s a core part of the software development process, especially in modern development practices like Test-Driven Development (TDD) and Agile.
2. Types of unit testing
Unit testing can be performed in two main ways: manually or automatically. Each approach has its own strengths and is suitable for different scenarios depending on the project’s needs, size, and resources.
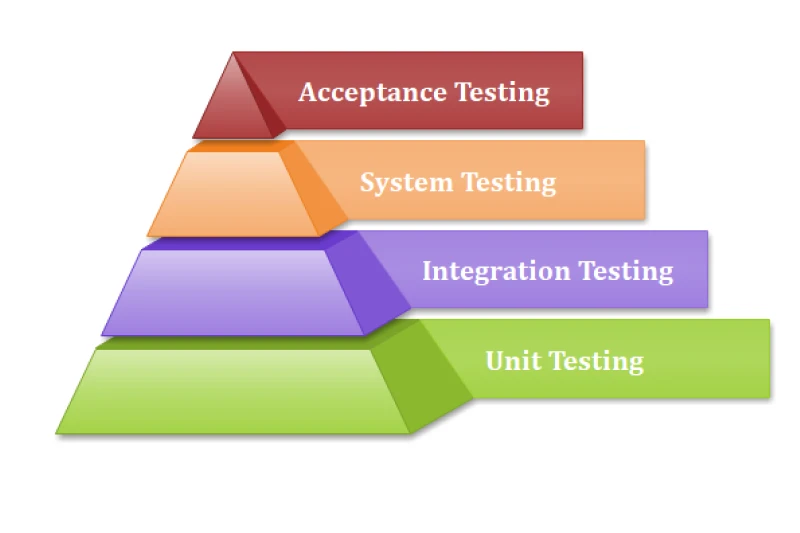
Feature
|
Manual Unit Testing
|
Automated Unit Testing
|
Speed
|
Slow
|
Fast and scalable
|
Reliability
|
Prone to human error
|
Consistent and repeatable
|
Maintenance effort
|
High
|
Low once set up
|
Best for
|
Simple or one-off tests
|
Ongoing, large-scale projects
|
2.1. Manual unit testing
Manual unit testing involves checking individual units of code by hand, typically by running the program andd verifying outputs against expected results. Developers often use print statements, simple test scripts, or even visual inspection to ensure the code behaves as intended.
This approach can be useful in small projects, quick prototypes, or when testing simple logic that doesn’t require complex setups. However, manual testing is time-consuming and not ideal for scaling. It’s also more prone to human error, especially as the codebase grows and becomes harder to manage manually.
Read more >>> 19 Types of Manual Testing in Software Testing [Update 2025]
2.2. Automated unit testing
Automated unit testing is where things get smart. Instead of manually checking each function, developers write test cases that can be run automatically, often with just a single command. These tests validate the behavior of code in a repeatable and consistent way.
The benefits are huge: faster feedback, higher accuracy, and better integration with modern development practices like continuous integration (CI) and test-driven development (TDD). Tools like JUnit, TestNG, PyTest, Jest, and NUnit make it easy to write, organize, and run these tests across different programming languages and environments.
While manual testing might still have a place in quick validations or early-stage code, automated unit testing is the gold standard for modern software development. It saves time, reduces bugs, and gives developers peace of mind when making changes.
Read more >>>> 4 Difference Between Software Testing and Quality Assurance
3. Benefits of unit testing
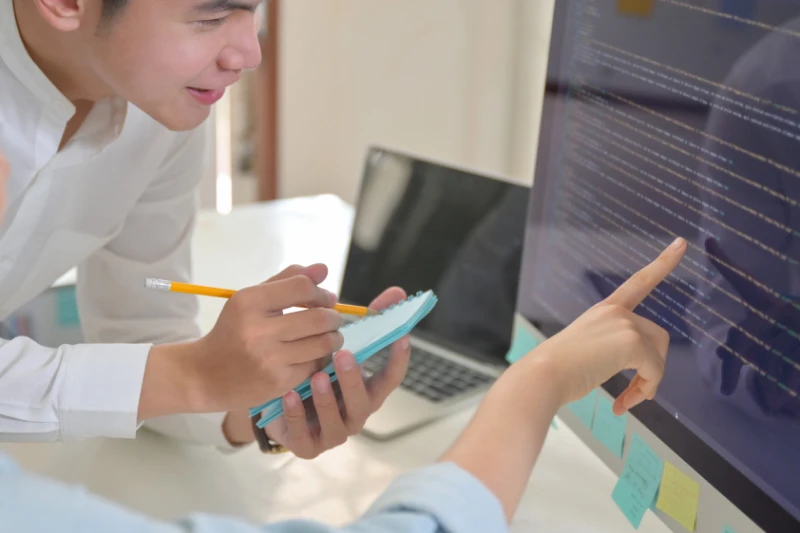
Unit testing isn’t just a box to check, it’s a game-changer in how we build and maintain software. Here are some of the biggest benefits it brings to the table:
- Early Detection of Bugs: One of the most powerful advantages of unit testing is its ability to catch bugs early, often before they even make it past your development machine. By testing small pieces of code in isolation, developers can identify issues at the root, long before they grow into bigger, costlier problems.
- Improved Code Quality and Maintainability: Clean code isn’t just about style, it’s about reliability. Unit tests encourage developers to write modular, well-structured functions that are easier to test, debug, and maintain. Over time, this leads to better software architecture and fewer headaches down the line.
- Facilitates Refactoring: Refactoring is a normal part of evolving any codebase, but it can be scary without the right safety net. With solid unit tests in place, developers can confidently update, optimize, or rewrite code, knowing that the tests will quickly flag anything that breaks in the process.
- Enhances Developer Confidence: There’s nothing more reassuring than instant feedback. Unit tests give developers the confidence to push changes, fix bugs, or add new features without fear of unexpected consequences. It’s like having a second set of eyes watching over every line you write.
- Provides Documentation Through Tests: Unit tests also serve as live documentation. Instead of digging through pages of outdated docs, developers can simply read the tests to understand what a piece of code is supposed to do. This makes onboarding new team members faster and helps everyone stay aligned with functionality.
4. How unit testing works
So, how does unit testing actually happen in real-world projects? Let’s break it down step by step—from writing the first test to full CI/CD integration.
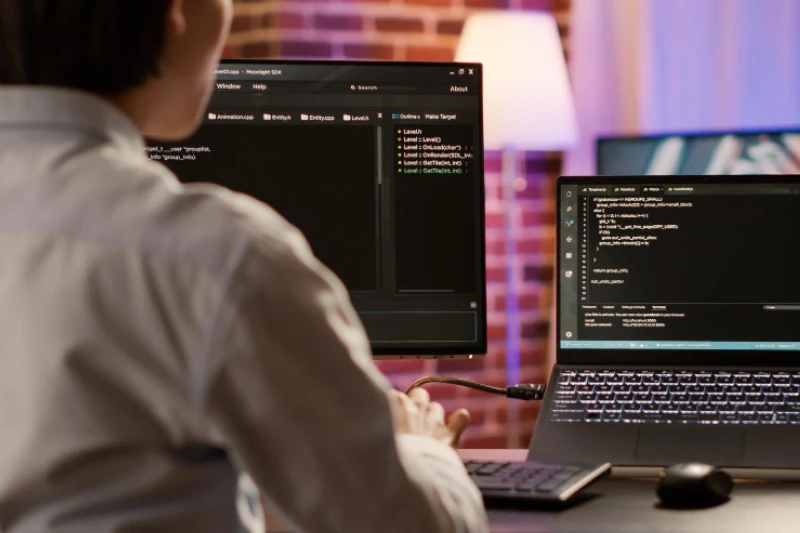
4.1. Writing unit tests
Everything starts with writing simple, focused test cases. A unit test targets a single function, method, or class and verifies that it produces the correct output for a given input. Developers typically use a testing framework, like JUnit for Java or PyTest for Python, to define these tests in a consistent format.
Good unit tests are:
- Small and independent
- Easy to read and understand
- Named clearly to reflect the purpose of the test
4.2. Executing tests
Once written, tests are executed using the test runner provided by the framework. This can be done manually from the command line, integrated into an IDE, or triggered automatically. During execution, the framework checks if the actual output matches the expected result for each test.
If everything passes, awesome. If not, the framework will point out exactly which test failed, helping developers pinpoint the problem fast.
4.3. Interpreting results
The output from a test run typically includes:
- Pass/fail status
- Error messages or stack traces for failed tests
- Code coverage metrics (if enabled)
These results help teams assess the health of the code. A failing test indicates either a bug in the code or a change that wasn’t accounted for—both of which need attention before moving forward.
4.4. Integrating with CI/CD pipelines
To take unit testing to the next level, it’s integrated into CI/CD pipelines. Tools like Jenkins, GitHub Actions, Travis CI, or GitLab CI automatically run unit tests every time code is pushed to a repository.
This automation ensures that:
- Broken code never makes it into production
- Bugs are caught early in the development cycle
- Teams maintain high-quality standards without slowing down delivery
With CI/CD, unit testing becomes a seamless part of the workflow, giving teams fast feedback and enabling continuous delivery with confidence.
5. Popular unit testing frameworks
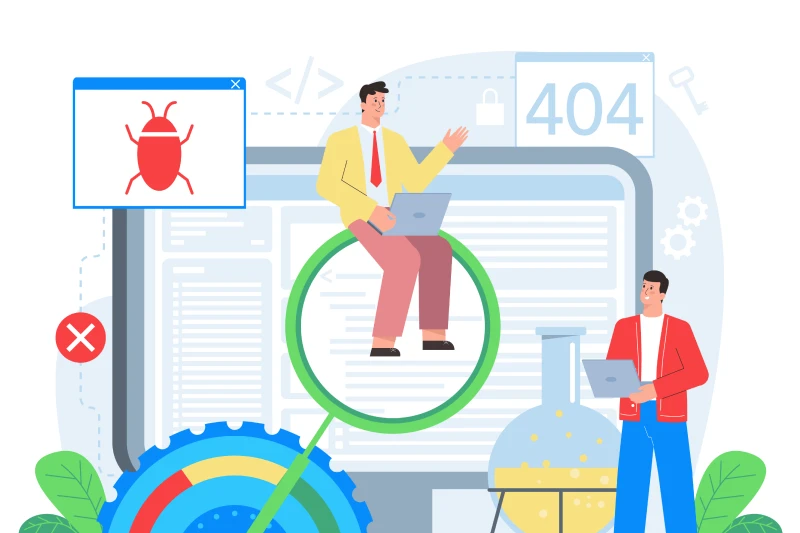
Choosing the right unit testing framework can make a huge difference in your development workflow. Here are some of the most widely used and trusted tools across different programming languages:
5.1. JUnit (Java)
A long-standing favorite in the Java ecosystem, JUnit provides annotations, assertions, and test runners to make unit testing seamless. It’s lightweight, fast, and integrates well with tools like Maven and Jenkins.
Key features:
- Easy test lifecycle management
- Strong support for parameterized tests
- Integration with CI/CD pipelines and building tools
5.2. TestNG (Java)
Inspired by JUnit but with more powerful configuration capabilities, TestNG is great for writing flexible, maintainable tests. It’s ideal for both unit and integration testing.
Key features:
- Grouping and prioritizing tests
- Data-driven testing with XML configuration
- Better parallel test execution
5.3. NUnit (.NET)
For developers working in the .NET ecosystem, NUnit is a top choice. It’s a mature, open-source testing framework that works well with C# and integrates smoothly with Visual Studio.
Key features:
- Rich set of assertions
- Custom test attributes
- Easy integration with .NET build pipelines
5.4. PyTest (Python)
Loved by the Python community for its simplicity and power, PyTest supports everything from small unit tests to complex functional testing.
Key features:
- Minimal boilerplate code
- Fixture system for reusable setup logic
- Plugins for extended functionality
5.5. Jest (JavaScript/TypeScript)
Developed by Facebook, Jest is the go-to testing framework for JavaScript and React applications. It’s fast, easy to use, and comes bundled with great default configurations.
Key features:
- Snapshot testing for UI components
- Built-in mocking capabilities
- Zero-configuration setup for most projects
6. Best practices for effective unit testing
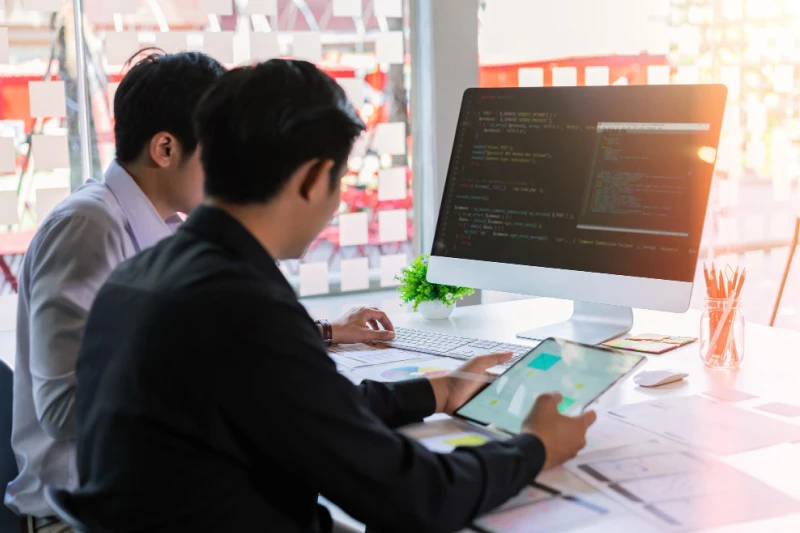
Writing unit tests isn’t just about getting them to pass—it’s about writing them well. Here are some golden rules that make your tests reliable, maintainable, and genuinely useful:
6.1. Write clear and concise tests
Each test should focus on a single behavior and have a clear purpose. Use descriptive test names that explain what the test is doing and why it matters. Avoid overcomplicating logic within the test itself—clarity wins over cleverness.
- Good: testUserLogin_WithValidCredentials_ReturnsSuccess()
- Bad: test123()
6.2. Maintain test independence
Tests should never depend on the order in which they run or the results of other tests. If one test fails, it shouldn’t affect the outcome of others. Keeping tests isolated ensures more consistent and trustworthy results.
6.3. Ensure tests are repeatable and reliable
A test that passes once but fails later for no clear reason is worse than no test at all. Make sure your tests don’t rely on external systems (like live APIs or databases) unless properly mocked. They should always give the same result under the same conditions.
6.4. Mock dependencies appropriately
When a function interacts with external components, like databases, APIs, or file systems, mocking those dependencies helps you focus on just the unit being tested. Tools like Mockito (Java), unittest.mock (Python), or Jest mocks (JavaScript) make this easy and effective.
7. Conclusion
In the fast-paced world of software development, quality and reliability are non-negotiable, and that’s exactly where unit testing comes in. If you’re still wondering what is unit testing in software testing, it’s a method of testing individual components of your code to ensure they work as intended.
From catching bugs early to enabling fearless refactoring, unit testing provides a strong foundation for building scalable, maintainable, and robust software systems. Whether you’re just starting out or leading a development team, adopting unit testing practices is a smart move that pays off in both the short and long term.
Start small, test often, and watch your codebase transform into something you can truly trust.