JavaScript is essential for web development, but dynamic typing in JavaScript can lead to unexpected errors, especially in large-scale projects. Debugging and maintenance become harder as codebases grow.
TypeScript, a superset of JavaScript, solves this with static typing in TypeScript, type inference, and interfaces. It helps catch errors early, improves readability, and makes scaling easier. If you’re deciding between TypeScript vs JavaScript, understanding their differences can help you choose the right tool for your project.
1. TypeScript vs JavaScript syntax differences
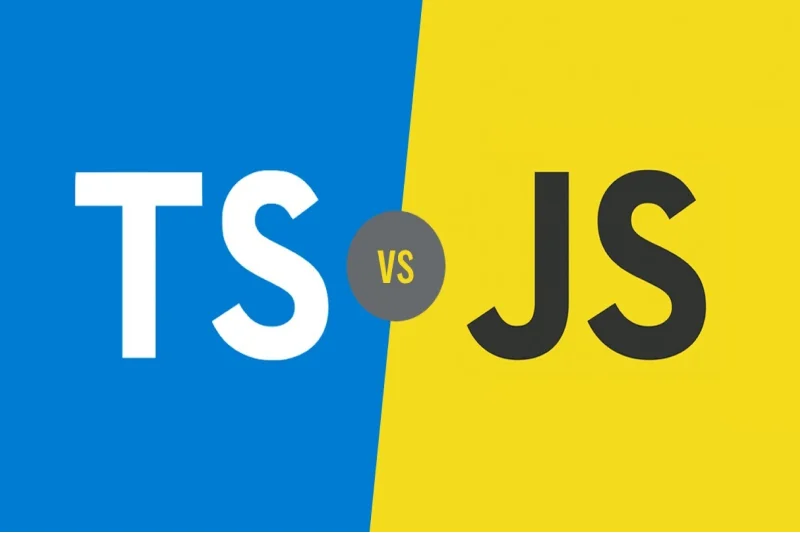
JavaScript’s flexibility comes with the downside of dynamic typing, which can lead to errors in large projects. TypeScript enhances JavaScript syntax by introducing static typing, interfaces, and strict null checks, making code safer and more maintainable.
Feature
|
JavaScript (Dynamic)
|
TypeScript (Static)
|
Variable declarations
|
No fixed data types, can change anytime
|
Requires defining types like number, string, boolean
|
Function parameters
|
Accepts any type of input, which can lead to errors
|
Requires specifying input types and output types to prevent mistakes
|
Classes & interfaces
|
Uses classes but lacks structure enforcement
|
Supports interfaces to organize code better
|
Null & undefined
|
Can cause unexpected issues
|
Strict null checks prevent common errors
|
1.1. Variables and type declarations
In JavaScript, you can assign a value to a variable without specifying its type. Later, the same variable can hold a different type of value, which may cause unexpected issues. TypeScript solves this by enforcing static typing, ensuring a variable only holds one value type.
1.2. Function and method definitions
JavaScript allows functions to take any kind of input, which can lead to unintended errors. In TypeScript, you must define what type of values the function should receive and return. This makes code clearer and more predictable.
1.3. Classes and interfaces in TypeScript
Both JavaScript and TypeScript support object-oriented programming (OOP). However, TypeScript introduces interfaces, which act as blueprints to ensure consistency in code structure. This is especially helpful in large projects where multiple developers work on the same codebase.
1.4. Handling null/undefined
In JavaScript, if a value is “null” or “undefined”, trying to use it can cause errors. TypeScript prevents this issue by enforcing strict null checks, ensuring developers handle such cases properly.
The differences between TypeScript and JavaScript for beginners mainly come down to structure and reliability. TypeScript features help reduce errors, improve code quality, and make large projects easier to maintain. For complex applications, many developers find TypeScript over JavaScript to be the better choice.
2. Why TypeScript is better than JavaScript?
When comparing TypeScript vs JavaScript, TypeScript offers several advantages that make it a better choice, especially for large projects. When considering why use TypeScript over JavaScript, its static typing and structured approach help prevent errors and improve long-term scalability.
2.1. Static typing and type inference
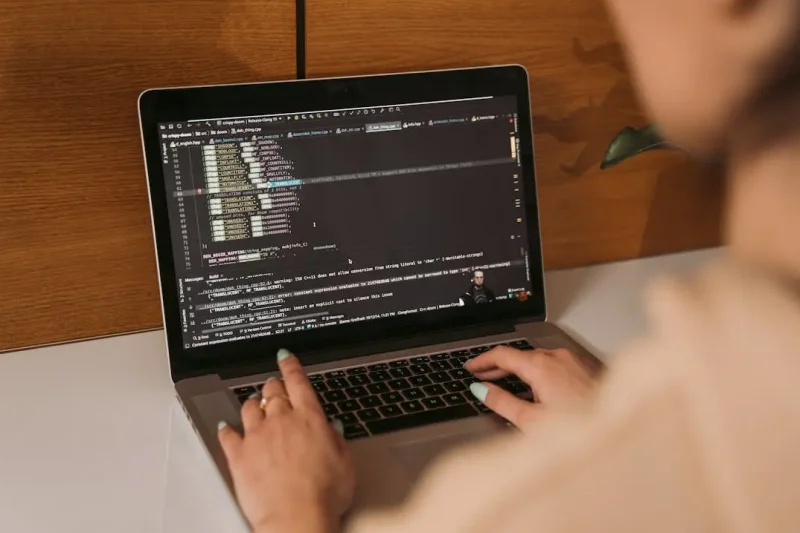
One of the biggest benefits of TypeScript features is static typing. Unlike JavaScript, where variable types can change unexpectedly, TypeScript enforces type consistency. This leads to:
- Fewer errors during development.
- Clearer, more readable code that’s easier to maintain.
- Better auto-completion and refactoring in IDEs like Visual Studio Code.
Additionally, TypeScript’s type inference automatically detects variable types without explicit annotations, reducing manual work while keeping code safe.
2.2. Error detection and prevention at compile-time
In JavaScript, errors often appear at runtime, making bugs hard to track. TypeScript’s compiler catches mistakes before execution, preventing unexpected crashes and saving debugging time. This is especially useful for large-scale applications, where errors can be costly.
2.3. Enhanced code readability and maintenance
With TypeScript syntax, developers can define types for variables, functions, and objects, making the code self-explanatory. This improves collaboration, especially in teams, as others can easily understand the structure of the code without extra documentation.
2.4. Better for large-scale applications
For large projects, managing complex JavaScript frameworks vs TypeScript frameworks can be challenging. TypeScript’s interfaces and strict type-checking help organize code, making it scalable and maintainable. Many companies use TypeScript over JavaScript for enterprise applications, where stability and reliability are crucial.
While JavaScript remains a powerful language, TypeScript’s static typing, compile-time error detection, and structured approach make it a better choice for developers who want to build reliable, scalable applications.
Read more >>> What Is the Difference Between Flask and Django?
3. TypeScript vs JavaScript code comparison
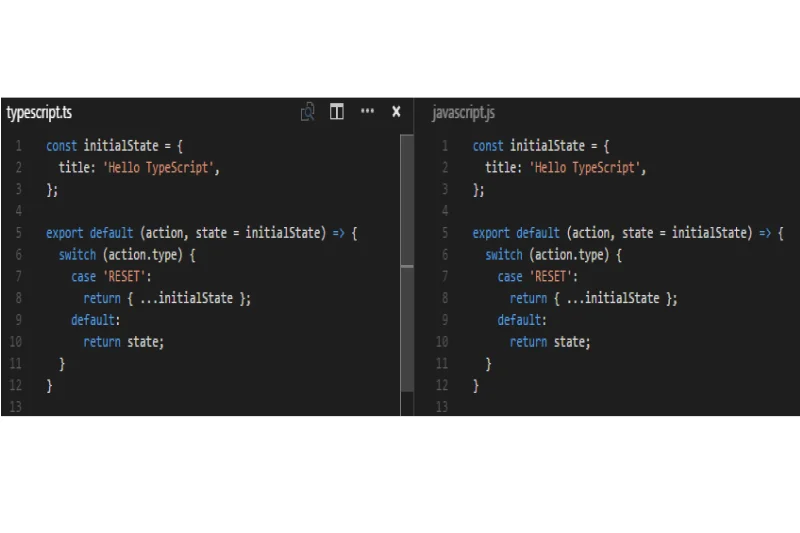
TypeScript vs JavaScript code comparison shows that the biggest difference is static typing. JavaScript offers flexibility but can cause unexpected errors. TypeScript improves reliability with type safety, reducing bugs and making code more predictable.
3.1. Variable Declarations
In JavaScript, you can assign any value to a variable, and it can change type later. This flexibility can cause errors.
- JavaScript (Dynamic Typing)
- You don’t need to specify the type.
- A variable can hold any type of value.
Example:
let message = “Hello”;
message = 123; // No error, but this might cause issues later.
- TypeScript (Static Typing)
- You must define the variable type (string, number, boolean).
- The value cannot change to a different type.
Example:
let message: string = “Hello”;
message = 123; // Error: Type ‘number’ is not assignable to type ‘string’.
- Why is this better?
With TypeScript features, errors are detected early, preventing mistakes before running the code.
3.2. Functions and Return Types
In JavaScript, functions do not enforce input types. This means incorrect values can be passed, leading to unexpected results.
- JavaScript (No Type Safety)
Example:
function add(a, b) {
return a + b;
}
console.log(add(5, “10”)); // Output: “510” (Unexpected result)
- TypeScript (Strict Type Checking)
Example:
function add(a: number, b: number): number {
return a + b;
}
console.log(add(5, “10”)); // Error: Argument of type ‘string’ is not assignable to parameter of type ‘number’.
- Why is this better?
With TypeScript syntax, you define the expected input and output types, preventing errors before they happen.
3.3. Classes and Interfaces
In JavaScript, you can create classes, but there are no interfaces to enforce structure.
- JavaScript (No Interface Support)
Example:
class User {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
- TypeScript (Better Structure with Interfaces)
Example:
interface User {
name: string;
age: number;
}
class Person implements User {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
}
- Why is this better?
- TypeScript enforces a structured approach with interfaces, making it easier to manage large codebases.
- Helps prevent missing or incorrect properties when working in teams.
When looking at TypeScript vs JavaScript, TypeScript stands out with static typing, stricter error checking, and better structure. It helps developers write cleaner, more reliable code. If you want fewer bugs and a scalable project, switching to TypeScript over JavaScript is a great decision.
Read more >>> C# vs Java: Similarities, Differences, and Practical Insights
4. Pros and Cons of TypeScript vs JavaScript
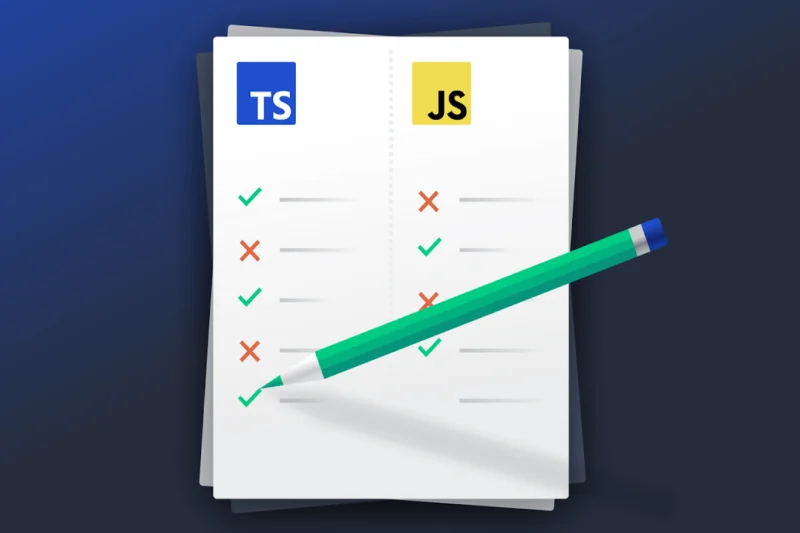
When comparing TypeScript vs JavaScript, both have strengths and weaknesses. TypeScript offers more structure and safety, while JavaScript provides flexibility and simplicity.
4.1. TypeScript development advantages
- Static typing prevents errors before execution, making code more reliable.
- Improves code quality with better readability and maintainability.
- Enhances developer productivity with code completion, type inference, and refactoring support in IDEs.
- Ideal for large-scale applications, ensuring better organization and fewer bugs.
- Works well with JavaScript frameworks vs TypeScript frameworks like React, Angular, and Vue.js.
4.2. TypeScript development disadvantages
- Has a learning curve, especially for developers unfamiliar with static typing.
- Requires compilation, adding extra steps before running the code.
- It can be overhead for small projects that don’t need strict type safety.
4.3. JavaScript development advantages
- Highly flexible, allowing quick prototyping and fast development.
- Supported by a large community with extensive libraries and frameworks.
- No need for compilation, making it easier to run immediately in the browser.
- Widely used in front-end development and supported across all web platforms.
4.4. JavaScript development disadvantages
- Dynamic typing can lead to unexpected runtime errors, making debugging harder.
- Lacks structure in large projects, resulting in messy, difficult-to-maintain code.
- Without strict type checking, errors may go unnoticed until the program runs.
If you need scalability, error prevention, and better long-term maintainability, TypeScript over JavaScript is the better choice. However, for smaller projects, rapid development, or quick prototyping, JavaScript’s simplicity and flexibility may be more practical.
5. When should you use TypeScript over JavaScript?
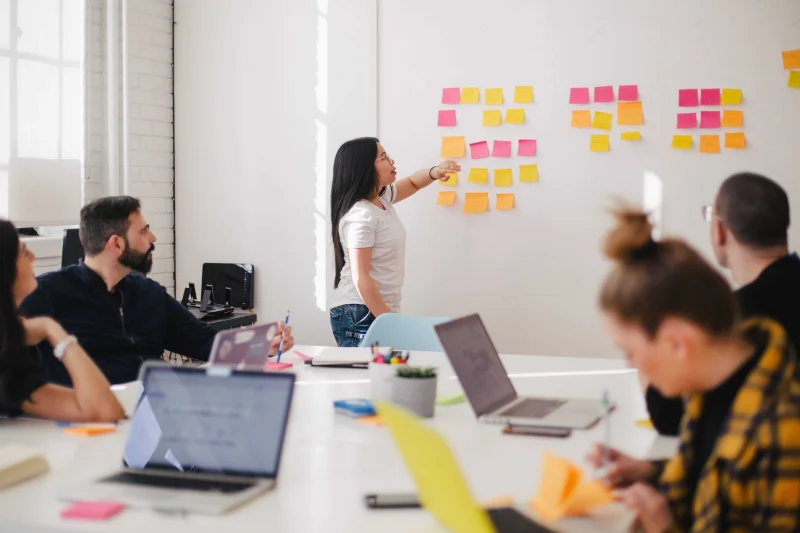
Choosing between TypeScript vs JavaScript depends on your project size, complexity, and long-term goals.
5.1. TypeScript for large projects and teams
For large-scale applications, TypeScript is a better choice. Its static typing, interfaces, and strict type checks help teams manage complex codebases. With multiple developers working on the same project, TypeScript features ensure consistency, reduce errors, and improve collaboration. It’s commonly used in enterprise software and JavaScript frameworks vs TypeScript frameworks like Angular, React, and Vue.js.
5.2. JavaScript for small, quick projects
If you’re working on a small project, JavaScript is often more practical. It’s lightweight, flexible, and doesn’t require compilation. For simple websites, prototypes, or quick experiments, JavaScript allows faster development without the setup required for TypeScript.
5.3. When to transition from JavaScript to TypeScript
If your project is growing and you’re facing maintainability, debugging, or scalability issues, it may be time to switch to TypeScript. The transition is smooth since TypeScript is a superset of JavaScript, meaning you can gradually introduce it without rewriting everything. Start by adding TypeScript to new files while keeping the existing JavaScript code intact.
6. How different is TypeScript from JavaScript?
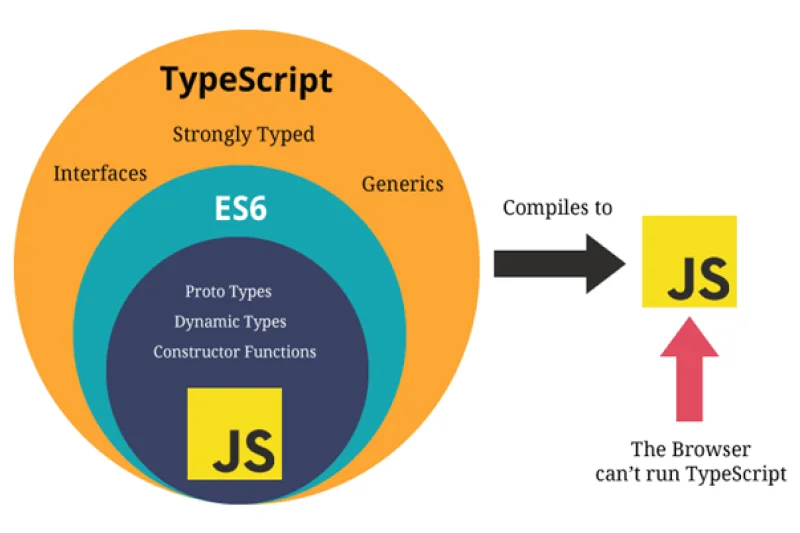
While TypeScript is built on JavaScript, it introduces new features that enhance development.
6.1. Language structure and features
The key difference between TypeScript vs JavaScript is static typing. In JavaScript, variables can change types anytime, leading to errors. TypeScript enforces strict type-checking, making code more predictable. It also supports interfaces, enums, and advanced OOP concepts, providing more structure.
6.2. Development environments and tooling
TypeScript integrates well with Visual Studio Code, WebStorm, and Atom. It offers better auto-completion, error detection, and debugging features than JavaScript, making development faster and smoother.
6.3. Compatibility with existing JavaScript code
Since TypeScript is a superset of JavaScript, existing JavaScript code can run without modification. This allows developers to adopt TypeScript gradually, making upgrading a project without major rewrites easy.
If you’re working on a small project, JavaScript is fine. But for scalability, reliability, and better team collaboration, TypeScript over JavaScript is the smarter choice.
7. Conclusion
TypeScript vs JavaScript is a common debate, but TypeScript stands out for large-scale applications. For small projects, JavaScript is fine. But TypeScript over JavaScript is the smarter choice for scalability and long-term stability. Start using TypeScript features today and improve your code.