Integration testing ensures software components work together seamlessly, a vital part of software testing. For software developers, QA engineers, and IT professionals, integration testing in software testing supports software quality assurance in continuous integration and CI/CD pipelines.
1. What is integration testing?
Integration testing in software testing is a crucial phase in software testing where individual modules or components of a software application are combined and tested as a group. The goal is to verify that these components work together seamlessly, ensuring the system functions as intended. Unlike unit testing, which focuses on testing individual pieces of code in isolation, integration testing checks the interactions and data flow between modules. It’s like making sure all the pieces of a puzzle fit together perfectly to form the complete picture.
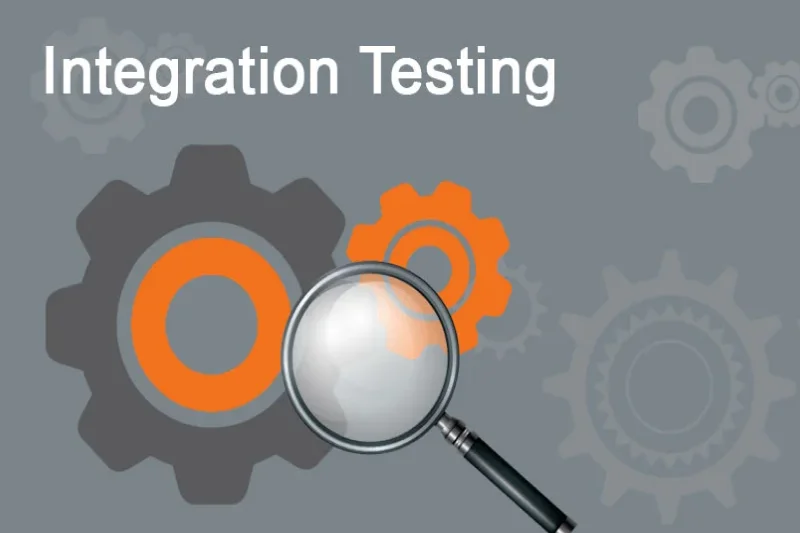
In software development, applications are often built as a collection of smaller, independent modules – think of them as building blocks. These could be functions, classes, or services like APIs or microservices. While each block may work fine on its own, problems can arise when they’re combined. Integration testing ensures that these interactions don’t lead to errors, miscommunications, or unexpected behavior.
Read more >>> 4 Difference Between Software Testing and Quality Assurance
2. Importance of integration testing in software development
What is integration testing in software development? It’s the process that bridges the gap between isolated component testing and full system testing. Without it, you might have perfectly functioning individual parts but a system that fails when those parts try to “talk” to each other. For example, imagine a web application where the user interface is flawless, but it fails to retrieve data from the backend due to a communication error. Integration testing in software testing catches these issues early.
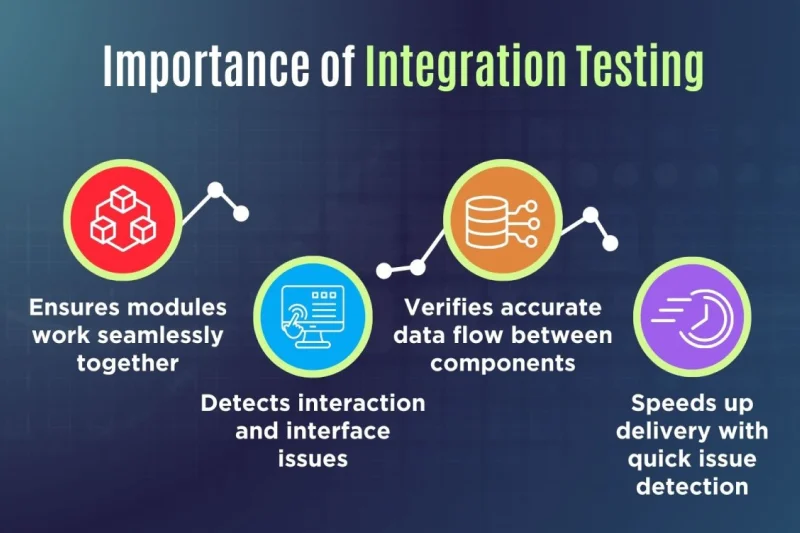
The significance of integration testing lies in its ability to improve software quality assurance. It reduces the risk of defects slipping into production, enhances system reliability, and ensures a smoother user experience. By identifying issues in how components interact, it saves time and cost compared to fixing problems later in the software development lifecycle. Plus, it supports practices like continuous integration, where code changes are frequently merged and tested, ensuring the system remains stable as it evolves.
3. Types of integration testing
Integration testing in software testing comes in several flavors, each suited to different project needs. Let’s explore the main types of integration testing in software.
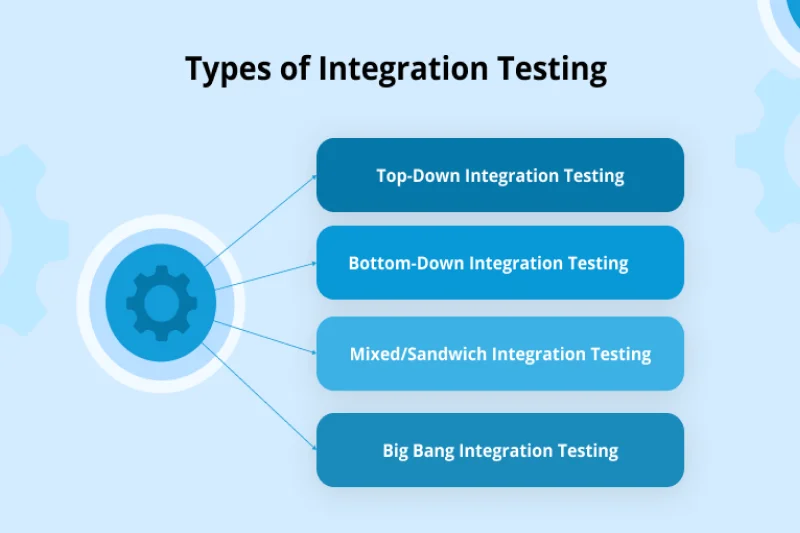
3.1. Big bang integration testing
In big bang integration testing, all modules are integrated and tested together in one go. It’s like assembling an entire machine and then turning it on to see if it works. This approach is simple but risky—finding the root cause of a failure can be tricky when everything is tested at once. It’s best suited for smaller projects with fewer components, where the complexity of interactions is manageable.
3.2. Incremental integration testing
Incremental integration testing takes a more gradual approach, combining and testing modules step by step. This method is more controlled and makes it easier to pinpoint issues. It’s divided into three main approaches:
Top-down approach: The top-down approach starts testing from the higher-level modules (like the user interface) and works downward to the lower-level ones (like the database). If lower-level modules aren’t ready, developers use mocking and stubbing to simulate their behavior. This approach is great for ensuring the main functionalities are tested early but may delay testing of core components.
Bottom-up approach: In contrast, the bottom-up approach begins with the lower-level modules and moves up to the higher-level ones. This ensures foundational components, like database operations, are solid before testing the user-facing parts. It’s useful when core services are critical to the system, such as in a microservices architecture.
Sandwich approach: The sandwich approach (or hybrid approach) combines the best of both worlds, testing from both the top and bottom simultaneously and meeting in the middle. This balanced method is efficient for complex systems but requires careful coordination to manage dependencies.
Read more >>> 19 Types of Manual Testing in Software Testing [Update 2025]
4. Differences between Integration testing vs unit testing
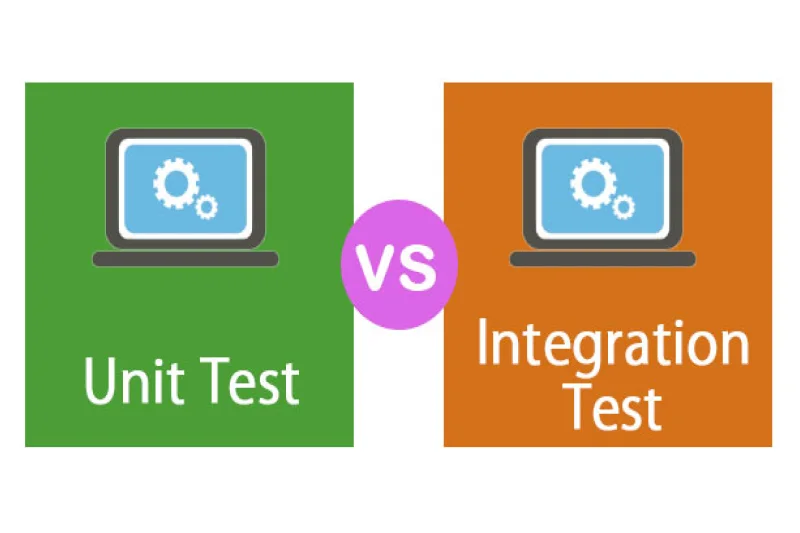
4.1. Key differences
Integration testing vs unit testing is a common point of comparison. Unit testing focuses on individual components—like a single function or class—in complete isolation, often using tools like JUnit for Java applications. It’s fast and precise but doesn’t account for how components interact. Integration testing, on the other hand, tests the connections between components, ensuring they work together as expected. For instance, unit testing might verify that a login function processes credentials correctly, while integration testing checks if the login function communicates properly with the authentication server.
The scope is another key difference. Unit testing is narrow, targeting small units of code, while integration testing has a broader focus, examining module interactions. Unit testing is typically done by developers during coding, whereas integration testing often involves QA engineers or software testers to validate system behavior.
4.2. When to use each type
Use unit testing early in development to catch bugs in individual components. It’s ideal for test-driven development (TDD), where tests are written before the code itself. Integration testing comes into play after unit testing, once components are ready to be combined. It’s critical in continuous integration/continuous deployment (CI/CD) pipelines, where frequent code integrations need to be validated to maintain system stability.
5. How integration testing works
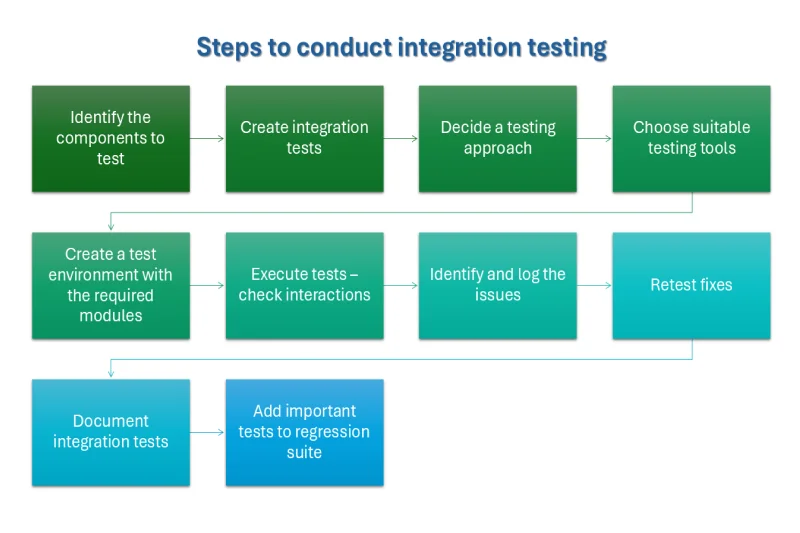
5.1. Integration testing process
How does integration testing work in software testing? The process typically follows these steps:
1. Plan the testing: Identify which modules to test, define test scenarios, and determine the order of integration (e.g., top-down, bottom-up, or sandwich approach).
2. Prepare test data: Create realistic data to simulate real-world interactions, such as API requests or database queries.
3. Integrate modules: Combine the components, either all at once (big bang) or incrementally.
4. Execute tests: Run test cases to verify interactions, using integration testing tools like Selenium for web applications or Postman for API testing.
5. Analyze results: Check for errors, such as data mismatches or communication failures, and debug as needed.
6. Repeat: Continue testing as more modules are integrated or code changes are made, often in a CI/CD pipeline managed by tools like Jenkins.
This process ensures that the system behaves as expected when components are combined, catching issues that unit testing might miss.
5.2. Tools and frameworks for integration testing
Integration testing tools make the process more efficient and reliable. Here are some popular ones:
- JUnit: A go-to framework for Java developers, JUnit supports both unit testing and integration testing by allowing testers to write test cases for module interactions.
- Selenium: Perfect for automated integration testing of web applications, Selenium automates browser actions to test user interfaces and their backend connections.
- Postman: Widely used for API testing, Postman lets testers send requests to APIs and verify responses, ensuring services in a microservices architecture communicate correctly.
- Jenkins: An automation server that integrates integration testing into CI/CD pipelines, enabling teams to run tests automatically whenever code is updated.
Automated integration testing is a game-changer, saving time and ensuring consistency. By automating repetitive test cases, teams can focus on analyzing results and improving the system.
5.3. Best practices in integration testing
To get the most out of integration testing, follow these best practices:
- Start early: Integrate and test components as soon as they’re ready to catch issues early in the software development lifecycle.
- Use realistic test data: Simulate real-world scenarios to ensure tests reflect actual usage.
- Leverage mocking and stubbing: When some modules aren’t ready, use mocking and stubbing to simulate their behavior and keep testing on track.
- Automate where possible: Automated integration testing reduces human error and speeds up the process, especially in CI/CD environments.
- Prioritize test coverage: Ensure all critical interactions between modules are tested to minimize the risk of defects.
- Document everything: Keep clear records of test cases, results, and issues to streamline debugging and future testing.
These practices help software developers, QA engineers, and technical leads deliver high-quality software with fewer surprises.
6. Conclusion
Integration testing in software testing plays a pivotal role in software quality assurance. By ensuring that different modules work together seamlessly, it reduces the risk of failures in production and enhances the overall reliability of the system. Whether you’re building a simple app or a complex microservices architecture, integration testing is essential for delivering a product that meets user expectations.
Incorporating integration testing into a CI/CD pipeline, supported by tools like Jenkins, Selenium, Postman, and JUnit, ensures that code changes don’t break existing functionality. It’s a key step in creating robust, user-friendly software that stands up to real-world demands.