Kotlin and Java are two of the most widely used programming languages, particularly in Android development. While Java programming has been the dominant language for decades, Kotlin programming has gained significant traction since Google officially declared it a preferred language for Android development in 2017.
The difference between Kotlin and Java lies in their syntax, functionality, and efficiency, making it essential for developers to understand their strengths and weaknesses before choosing the right language for their projects.
1. What is Kotlin?
Kotlin is a contemporary programming language with static typing that JetBrains created. Designed to be fully interoperable with Java programming, Kotlin programming eliminates many of Java programming’s common pain points, such as verbosity and null safety.
Understanding Java programming vs Kotlin’s advantages is crucial, especially for new developers who are considering which language to learn.
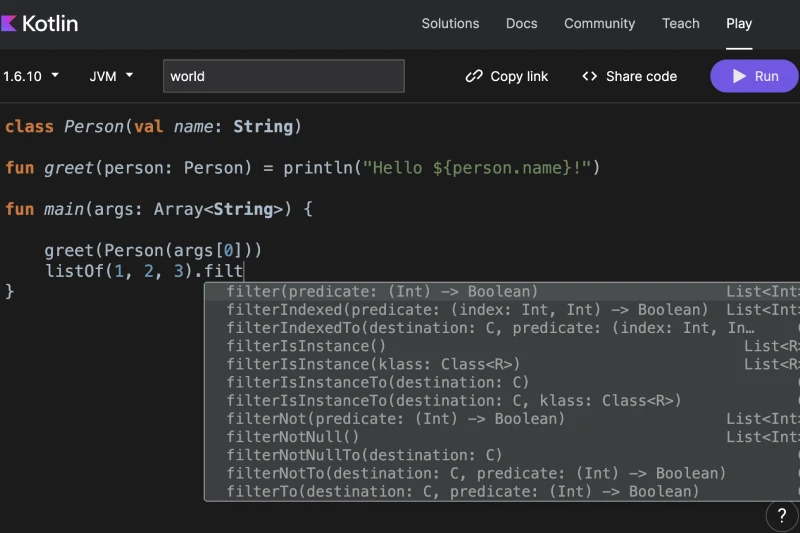
1.1. Kotlin features
Kotlin offers several modern features that enhance developer productivity and code safety.
- Minimizing null-related errors: Kotlin’s design incorporates null safety, effectively decreasing the risk of NullPointerExceptions, which are frequent errors in Java.
- Extending class functionality: Kotlin allows developers to augment the capabilities of existing classes through extension functions without altering the original class code.
- Streamlined asynchronous tasks: Kotlin’s Coroutines feature makes writing and managing code that performs operations concurrently easier.
- Reduced code volume: Kotlin’s syntax is more compact, requiring fewer lines of code than Java programming for the same tasks.
- Simplified data storage classes: Creating classes specifically for holding data is made more straightforward with Kotlin’s Data Classes.
1.2. Common applications of Kotlin:
Kotlin’s versatility makes it suitable for a range of development projects.
- Android app creation: Kotlin vs Java for Android is a common debate, but Kotlin is widely adopted as a primary language for building Android development mobile applications.
- Web application development: The Ktor framework enables Kotlin programming to create web-based applications.
- Server-side programming: Kotlin programming can be used for backend development, with strong integration with frameworks like Spring Boot.
- Building mobile apps for multiple platforms: Kotlin features over Java include Kotlin Multiplatform, which allows developers to share code between different mobile operating systems, facilitating Java vs Kotlin for mobile development.
Read more >>> Ruby vs PHP: Which Language is Best for Web Development?
2. What is Java?
Java, created by Sun Microsystems (now owned by Oracle), is one of the oldest and most widely used programming languages. It is known for its platform independence and robustness.
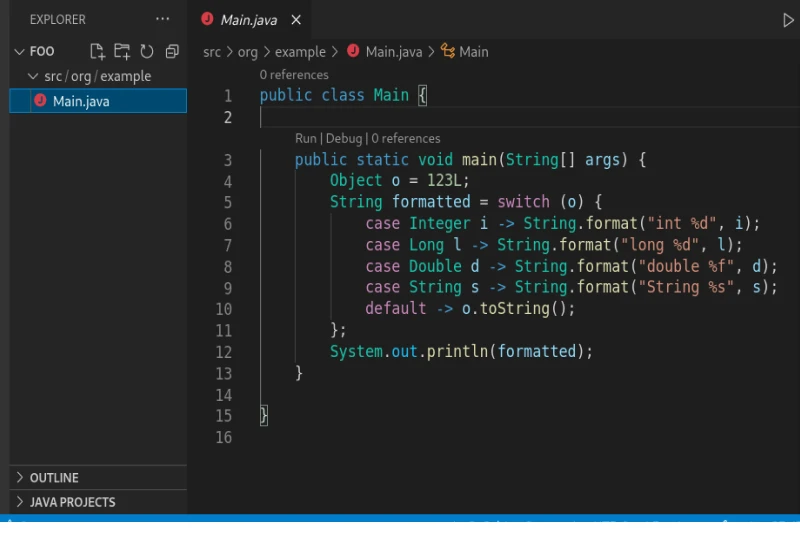
2.1. Java features
Java’s core features have contributed to its widespread adoption in enterprise environments.
- Object-oriented design: Java programming utilizes OOP principles, allowing code organization through encapsulation, inheritance, and polymorphism.
- Robust error management: Using checked exceptions enforces proactive error handling during development.
- Powerful concurrent processing: Java programming provides strong built-in support for multithreading, enabling efficient concurrent programming.
- Extensive development resources: A large and active community contributes to a vast ecosystem of libraries and frameworks.
- Platform versatility: Java programming’s JVM (Java Virtual Machine) compatibility allows it to run on various operating systems.
Read more >>> TOP 12 Best Java Machine Learning Libraries 2025
2.2. Common applications of Java:
Java’s robustness makes it the go-to language for many large-scale applications.
- Large-scale business systems: Java is frequently used to build complex enterprise applications in sectors like banking and e-commerce.
- Web application development: Java is a mainstay for web development, particularly with frameworks such as Spring and Java EE.
- Android app development (historically): Java was the dominant language for Android development before Kotlin gained widespread adoption.
- Data-intensive applications: Java is well-suited for big data and machine learning tasks, and it is often used with technologies like Hadoop and Apache Spark.
Read more >>> C# vs Java: Similarities, Differences, and Practical Insights
3. 10 Difference between Kotlin and Java
Kotlin vs. Java presents distinct approaches to coding, and understanding their differences is crucial.
3.1. Syntax differences
Understanding the difference between Kotlin and Java is essential for developers to choose the best language for their projects. Both have distinct syntax and functional approaches.
Kotlin programming provides a more concise syntax than Java programming. Here’s how both languages implement a basic “Hello, World!” program:
Java:
class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
Kotlin:
fun main() {
println(“Hello, World!”)
}
Kotlin eliminates redundant code, allowing for faster development and improved readability. Therefore, considering why Kotlin is more efficient than Java is a good idea for any new android project.
3.2. Null safety
One of the key Kotlin features is null safety, which helps prevent the common NullPointerException found in Java programming.
Java:
String name = null; // May trigger a NullPointerException
Kotlin:
var name: String? = null // Supports safe null handling
By enforcing null safety, Kotlin ensures fewer runtime crashes compared to Java.
3.3. Extension functions
A notable aspect of Kotlin programming is Extension Functions, which enable developers to improve existing classes without altering them.
Kotlin:
fun String.firstLetterUpperCase(): String {
return this.replaceFirstChar { it.uppercaseChar() }
}
With this function, any string can be transformed to start with an uppercase letter.
This feature makes code reuse easier compared to Java, which does not have built-in support for extension functions.
3.4. Code structure and data classes
Another advantage of Kotlin vs Java is Kotlin features over Java, such as data classes, which eliminate the need to manually create getter and setter methods.
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Kotlin:
data class User(val name: String)
Kotlin reduces unnecessary code using data classes, making the development process faster.
3.5. Coroutines for asynchronous programming
Kotlin introduces Coroutines, making concurrent programming easier than Java’s traditional thread-based approach.
Java (Thread-based approach):
Thread thread = new Thread(() -> System.out.println(“Executing in a new thread”));
thread.start();
Kotlin (Coroutines):
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch {
println(“Running in a coroutine”)
}
}
Using Kotlin vs Java performance comparisons, we can see that Coroutines in Kotlin simplify asynchronous programming and optimize system resources better than Java’s multithreading.
3.6. Checked exceptions:
Kotlin and Java differ in their handling of checked exceptions.
- Java: Java programming mandates handling checked exceptions via “try-catch” blocks, requiring explicit error management.
- Kotlin: Kotlin programming does not enforce checked exceptions, providing more flexibility.
3.7. Functional programming:
Kotlin’s functional programming support is more extensive than Java’s.
- Kotlin: Kotlin programming offers enhanced support for functional programming paradigms featuring lambda expressions and extension functions.
- Java: Java programming has added some functional features but is less extensive than Kotlin programming.
3.8. Primitive types
Kotlin and Java handle primitive types differently.
- Java: Distinguishes between primitive data types (e.g., “int”, “char”) and objects.
- Kotlin: Treats all data as objects while optimizing the use of primitive types for performance behind the scenes.
3.9. Public fields
Kotlin and Java take different approaches to field access.
- Java: Permits the direct declaration of public fields within classes.
- Kotlin: Promotes encapsulation by favoring the use of properties over direct public field access.
3.10. Wildcard types
Kotlin and Java utilize different mechanisms for generic type variance.
- Java: Java programming employs wildcard types (“? extends T”, “? super T”) for generic type variance.
- Kotlin: Kotlin programming utilizes type projections (“out T”, “in T”) instead.
Read more >>> Podman vs. Docker: Key Differences & Compatibility Explained
4. Why choose Kotlin over Java (or vice versa)?
The choice between Kotlin and Java depends on specific project needs and priorities.
4.1. When to choose Kotlin
Modern development practices and specific use cases favor Kotlin.
- For Android app development: Kotlin is Google’s preferred language for modern Android application development.
- For writing shorter, more readable code: Kotlin’s syntax is designed to be more concise, reducing the amount of boilerplate code needed compared to Java.
- For enhanced code reliability: Kotlin’s built-in null safety features significantly decrease the likelihood of NullPointerExceptions, leading to more stable applications.
Want to Integrate Powerful IT Solutions into Your Business?
We provide tailored IT solutions designed to fuel your success. Let`s map out a winning strategy. Starting with a free consultation.
Contact Us4.2. When to choose Java
Java remains a strong choice for established and performance-critical applications.
- For large, complex business systems: Java is a leading choice for developing robust enterprise-level applications due to its long-established presence and mature ecosystem.
- For established web application frameworks: Java frameworks like Spring Boot are extensively used for building scalable and reliable web applications.
- For fine-tuned performance control: Java offers developers granular control over memory management and performance optimization, which can be crucial for performance-critical applications.
5. Conclusion
The difference between Kotlin and Java extends beyond syntax. Kotlin is modern, concise, and safe, making it ideal for Android and new projects. However, Java remains crucial for enterprise applications and legacy systems. Understanding the pros and cons of both languages allows developers and decision-makers to choose the best tool for their specific needs.